RAG Fusion Implementation:¶
A Guide to Advanced Retrieval-Augmented Generation
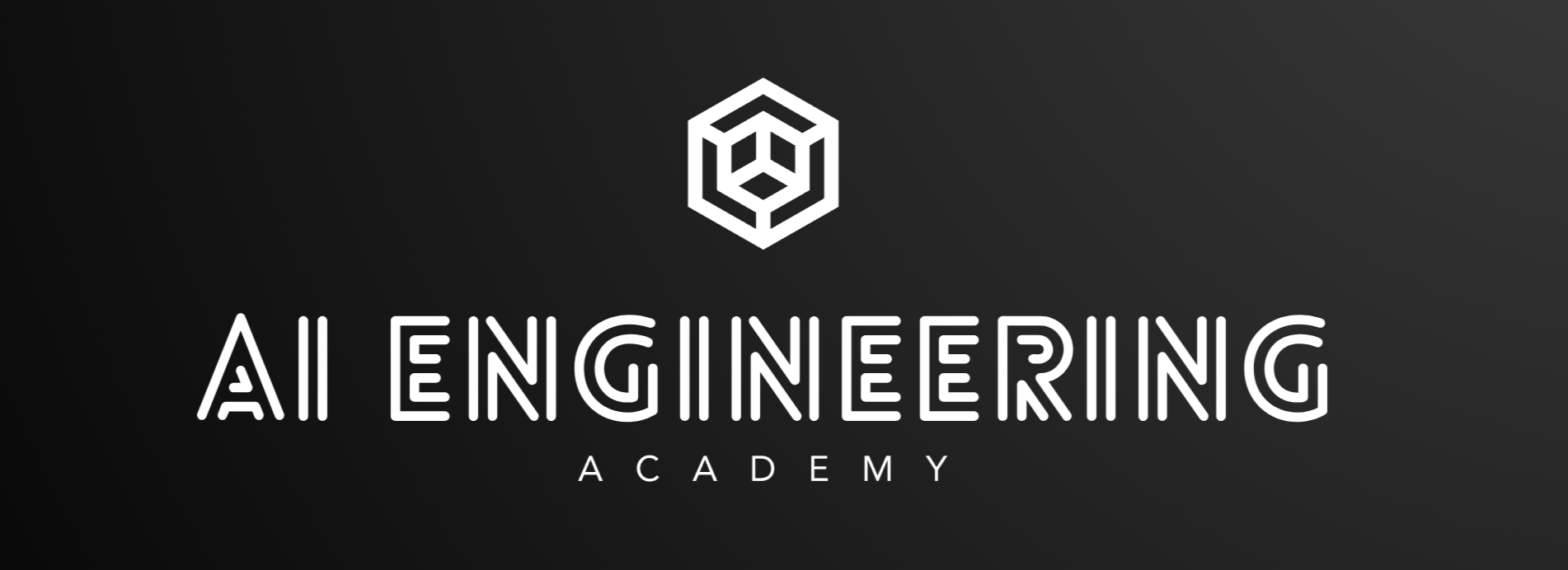
Welcome to the RAG Fusion Implementation guide! This notebook is designed to introduce you to the concept of RAG Fusion, an advanced technique that enhances the traditional Retrieval-Augmented Generation (RAG) approach. We'll provide a step-by-step walkthrough of implementing a RAG Fusion system.
Introduction¶
RAG Fusion is an advanced technique that builds upon the foundations of Retrieval-Augmented Generation (RAG). It combines the power of large language models with sophisticated information retrieval methods to produce more accurate, diverse, and contextually rich responses. RAG Fusion enhances the basic RAG approach by employing query expansion, multiple retrievals, and intelligent result combination techniques.
This notebook aims to provide a clear and comprehensive introduction to RAG Fusion, suitable for those who are familiar with basic RAG and want to explore more advanced implementations.
Getting Started¶
To get the most out of this notebook, you should have a good understanding of Python and be familiar with basic RAG concepts. Don't worry if some advanced ideas are new to you – we'll guide you through each step of the RAG Fusion process!
Prerequisites¶
- Python 3.9+
- Jupyter Notebook or JupyterLab
- Familiarity with basic RAG concepts
- Understanding of vector databases and embeddings
- Basic knowledge of natural language processing (NLP) concepts
Notebook Contents¶
Our notebook is structured into the following main sections:
Environment Set Up: We'll guide you through setting up your Python environment with all the necessary libraries and dependencies for RAG Fusion.
Query Expansion: Learn how to generate multiple queries from a single user input to capture different aspects of the user's intent.
Multiple Retrievals: Understand how to perform and manage multiple retrieval operations using various queries.
Reciprocal Rank Fusion (RRF): Dive into the RRF algorithm and how it's used to combine results from multiple retrievals effectively.
Context Selection and Reranking: Explore techniques for selecting diverse and relevant contexts from the fused retrieval results.
Enhanced Prompting: Learn how to craft effective prompts that leverage the diverse contexts obtained through RAG Fusion.
RAG Fusion Pipeline: We'll walk you through the process of setting up a complete RAG Fusion pipeline, integrating all the components.
Advanced Topics and Optimizations: Explore advanced concepts like dynamic query expansion, adaptive fusion techniques, and performance optimizations.
By the end of this notebook, you'll have a solid understanding of RAG Fusion and be able to implement this advanced technique in your own projects. Let's dive in and explore the cutting edge of Retrieval-Augmented Generation!
Set up environment¶
# Import necessary libraries
import os
from typing import List, Dict
from dotenv import load_dotenv
from IPython.display import Markdown, display
# OpenAI import
from openai import OpenAI
# LlamaIndex imports
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader, Document
from llama_index.core import Settings
from llama_index.vector_stores.qdrant import QdrantVectorStore
from llama_index.embeddings.fastembed import FastEmbedEmbedding
from llama_index.core.node_parser import SentenceSplitter
from llama_index.core.ingestion import IngestionPipeline
from llama_index.core.base.llms.types import ChatMessage, MessageRole
# Qdrant client import
import qdrant_client
# Load environment variables
load_dotenv()
# Get OpenAI API key from environment variables
OPENAI_API_KEY = os.getenv("OPENAI_API_KEY")
if OPENAI_API_KEY is None:
raise Exception("No OpenAI API key found. Please set it as an environment variable.")
# Initialize OpenAI client
client = OpenAI(api_key=OPENAI_API_KEY)
# Set the embedding model
Settings.embed_model = FastEmbedEmbedding(model_name="BAAI/bge-base-en-v1.5")
Function to generate queries using OpenAI's ChatGPT¶
def generate_queries_chatgpt(original_query: str) -> List[str]:
response = client.chat.completions.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant that generates multiple search queries based on a single input query."},
{"role": "user", "content": f"Generate multiple search queries related to: {original_query}"},
{"role": "user", "content": "OUTPUT (4 queries):"}
]
)
generated_queries = response.choices[0].message.content.strip().split("\n")
return generated_queries
Function to perform vector search¶
def vector_search(query: str, index: VectorStoreIndex) -> Dict[str, float]:
retriever = index.as_retriever(similarity_top_k=5)
nodes = retriever.retrieve(query)
return {node.node.get_content(): node.score for node in nodes}
Reciprocal Rank Fusion algorithm¶
def reciprocal_rank_fusion(search_results_dict: Dict[str, Dict[str, float]], k: int = 60) -> Dict[str, float]:
fused_scores = {}
print("Initial individual search result ranks:")
for query, doc_scores in search_results_dict.items():
print(f"For query '{query}': {doc_scores}")
for query, doc_scores in search_results_dict.items():
for rank, (doc, score) in enumerate(sorted(doc_scores.items(), key=lambda x: x[1], reverse=True)):
if doc not in fused_scores:
fused_scores[doc] = 0
previous_score = fused_scores[doc]
fused_scores[doc] += 1 / (rank + k)
print(f"Updating score for {doc} from {previous_score} to {fused_scores[doc]} based on rank {rank} in query '{query}'")
reranked_results = {doc: score for doc, score in sorted(fused_scores.items(), key=lambda x: x[1], reverse=True)}
print("Final reranked results:", reranked_results)
return reranked_results
Load the Data¶
reader = SimpleDirectoryReader("data", recursive=True)
documents = reader.load_data(show_progress=True)
documents = Document(text="\n\n".join([doc.text for doc in documents]))
Loading files: 100%|██████████| 1/1 [00:00<00:00, 8.30file/s]
Setting up Vector Database¶
We will be using qDrant as the Vector database There are 4 ways to initialize qdrant
- Inmemory
client = qdrant_client.QdrantClient(location=":memory:")
- Disk
client = qdrant_client.QdrantClient(path="./data")
- Self hosted or Docker
client = qdrant_client.QdrantClient(
# url="http://<host>:<port>"
host="localhost",port=6333
)
- Qdrant cloud
client = qdrant_client.QdrantClient(
url=QDRANT_CLOUD_ENDPOINT,
api_key=QDRANT_API_KEY,
)
for this notebook we will be using qdrant cloud
qdrant_client = qdrant_client.QdrantClient(location=":memory:")
vector_store = QdrantVectorStore(client=qdrant_client, collection_name="RAG_Fusion")
Ingest Data into vector DB¶
pipeline = IngestionPipeline(
transformations=[
SentenceSplitter(chunk_size=1024, chunk_overlap=20),
Settings.embed_model,
],
vector_store=vector_store,
)
nodes = pipeline.run(documents=[documents], show_progress=True)
print("Number of chunks added to vector DB:", len(nodes))
Parsing nodes: 100%|██████████| 1/1 [00:00<00:00, 193.16it/s] Generating embeddings: 100%|██████████| 2/2 [00:00<00:00, 2.51it/s]
Number of chunks added to vector DB: 2
Setting Up Retriever¶
# Create index
index = VectorStoreIndex.from_vector_store(vector_store=vector_store)
ChatEngineInterface with RAG Fusion¶
class ChatEngineInterface:
def __init__(self, index: VectorStoreIndex):
self.index = index
self.chat_history: List[ChatMessage] = []
def display_message(self, role: str, content: str):
if role == "USER":
display(Markdown(f"**Human:** {content}"))
else:
display(Markdown(f"**AI:** {content}"))
def chat(self, message: str) -> str:
user_message = ChatMessage(role=MessageRole.USER, content=message)
self.chat_history.append(user_message)
# Generate multiple queries
generated_queries = generate_queries_chatgpt(message)
# Perform vector search for each query
all_results = {}
for query in generated_queries:
search_results = vector_search(query, self.index)
all_results[query] = search_results
# Apply Reciprocal Rank Fusion
reranked_results = reciprocal_rank_fusion(all_results)
# Use reranked results to generate response
top_docs = list(reranked_results.keys())[:3] # Get top 3 documents
context = "\n".join(top_docs)
response = client.chat.completions.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant that answers questions based on the given context."},
{"role": "user", "content": f"Context:\n{context}\n\nQuestion: {message}"}
]
)
ai_response = response.choices[0].message.content.strip()
ai_message = ChatMessage(role=MessageRole.ASSISTANT, content=ai_response)
self.chat_history.append(ai_message)
self.display_message("USER", message)
self.display_message("ASSISTANT", ai_response)
print("\n" + "-"*50 + "\n") # Separator for readability
return ai_response
def get_chat_history(self) -> List[ChatMessage]:
return self.chat_history
# Usage
chat_interface = ChatEngineInterface(index)
# Example usage
chat_interface.chat("What is Samarth's CGPA?")
chat_interface.chat("What are all the AI projects done by samarth?")
Initial individual search result ranks: For query '1. Samarth CGPA transcript': {'PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern,': 0.7078029412854389, "Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.589905121981148, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.5303466536992336, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.5055852542659272, 'in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website.': 0.49850564846089873} For query '2. How to calculate CGPA for Samarth': {'PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern,': 0.6847357902557305, "Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.5511591679417467, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.46491744774645705, "| Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM": 0.45725453656695775, 'in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website.': 0.45656594020465413} For query '3. Samarth's college CGPA ranking': {'PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern,': 0.6937014902973797, "Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.5915607448451599, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.4915874323604704, 'Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.': 0.47546500691492116, "| Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM": 0.4713628999730835} For query '4. CGPA conversion scale for Samarth's university': {'PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern,': 0.696238816770899, "Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.5796498471210573, 'in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website.': 0.4964184623584387, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.4943453584081807, "| Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM": 0.48648555242994007} Updating score for PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, from 0 to 0.016666666666666666 based on rank 0 in query '1. Samarth CGPA transcript' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0 to 0.01639344262295082 based on rank 1 in query '1. Samarth CGPA transcript' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0 to 0.016129032258064516 based on rank 2 in query '1. Samarth CGPA transcript' Updating score for Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter.in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. It includes a custom-built hardware vending machine for immediate medicine dispensing Frequency Scaling + PWM Generator and 8 Bit UART Transmitter and Reciever iverilog implementation includes a frequency scaler with adjustable scaling factor and PWM generation based on a specified Duty Cycle. Additionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects Built a couple of Gen AI projects with a senior using preexisting diffusion models like StableDiffusionXL, InstantID, etc. for editing personalized video and cards. SmartGuardian www.gith ub.com/samarth777/smartguardian combines IoT with AI to create an autonomous car equipped with a virtual camera, allowing remote control and monitoring of surroundings with scene descriptions generated through AI integration MANET Research (ongoing) Researching Mobile Ad-hoc Networks under Dr. DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0 to 0.015873015873015872 based on rank 3 in query '1. Samarth CGPA transcript' Updating score for in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. from 0 to 0.015625 based on rank 4 in query '1. Samarth CGPA transcript' Updating score for PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, from 0.016666666666666666 to 0.03333333333333333 based on rank 0 in query '2. How to calculate CGPA for Samarth' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0.01639344262295082 to 0.03278688524590164 based on rank 1 in query '2. How to calculate CGPA for Samarth' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0.016129032258064516 to 0.03225806451612903 based on rank 2 in query '2. How to calculate CGPA for Samarth' Updating score for | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM from 0 to 0.015873015873015872 based on rank 3 in query '2. How to calculate CGPA for Samarth' Updating score for in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. from 0.015625 to 0.03125 based on rank 4 in query '2. How to calculate CGPA for Samarth' Updating score for PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, from 0.03333333333333333 to 0.05 based on rank 0 in query '3. Samarth's college CGPA ranking' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0.03278688524590164 to 0.04918032786885246 based on rank 1 in query '3. Samarth's college CGPA ranking' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0.03225806451612903 to 0.04838709677419355 based on rank 2 in query '3. Samarth's college CGPA ranking' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8. from 0 to 0.015873015873015872 based on rank 3 in query '3. Samarth's college CGPA ranking' Updating score for | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM from 0.015873015873015872 to 0.03149801587301587 based on rank 4 in query '3. Samarth's college CGPA ranking' Updating score for PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, from 0.05 to 0.06666666666666667 based on rank 0 in query '4. CGPA conversion scale for Samarth's university' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0.04918032786885246 to 0.06557377049180328 based on rank 1 in query '4. CGPA conversion scale for Samarth's university' Updating score for in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. from 0.03125 to 0.047379032258064516 based on rank 2 in query '4. CGPA conversion scale for Samarth's university' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0.04838709677419355 to 0.06426011264720942 based on rank 3 in query '4. CGPA conversion scale for Samarth's university' Updating score for | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM from 0.03149801587301587 to 0.04712301587301587 based on rank 4 in query '4. CGPA conversion scale for Samarth's university' Final reranked results: {'PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern,': 0.06666666666666667, "Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.06557377049180328, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.06426011264720942, 'in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website.': 0.047379032258064516, "| Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM": 0.04712301587301587, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.015873015873015872, 'Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.': 0.015873015873015872}
Human: What is Samarth's CGPA?
AI: Samarth's CGPA is 8.56 at PES University EC Campus where he is pursuing B. Tech CSE from 2022 to present.
-------------------------------------------------- Initial individual search result ranks: For query '1. "List of AI projects by Samarth"': {"Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.64229315400598, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.6300095332112591, 'ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.': 0.6282963988363128, 'DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6192984710699726, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6161929853493662} For query '2. "Samarth AI projects portfolio"': {'DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6299981396061565, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.629866091094448, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6285683327767199, "Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.6264142302163573, 'ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.': 0.617870348586077} For query '3. "Samarth artificial intelligence projects"': {"Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.6527829292498244, 'DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6331282865431569, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.6234571730754817, 'ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.': 0.6180030614680538, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6037578014381535} For query '4. "AI initiatives by Samarth"': {"Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.6422919010884514, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.6260785080263631, 'DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.6239354107056428, 'ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.': 0.6132845275349096, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.5978226655407697} Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0 to 0.016666666666666666 based on rank 0 in query '1. "List of AI projects by Samarth"' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0 to 0.01639344262295082 based on rank 1 in query '1. "List of AI projects by Samarth"' Updating score for ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter. from 0 to 0.016129032258064516 based on rank 2 in query '1. "List of AI projects by Samarth"' Updating score for DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0 to 0.015873015873015872 based on rank 3 in query '1. "List of AI projects by Samarth"' Updating score for Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter.in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. It includes a custom-built hardware vending machine for immediate medicine dispensing Frequency Scaling + PWM Generator and 8 Bit UART Transmitter and Reciever iverilog implementation includes a frequency scaler with adjustable scaling factor and PWM generation based on a specified Duty Cycle. Additionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects Built a couple of Gen AI projects with a senior using preexisting diffusion models like StableDiffusionXL, InstantID, etc. for editing personalized video and cards. SmartGuardian www.gith ub.com/samarth777/smartguardian combines IoT with AI to create an autonomous car equipped with a virtual camera, allowing remote control and monitoring of surroundings with scene descriptions generated through AI integration MANET Research (ongoing) Researching Mobile Ad-hoc Networks under Dr. DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0 to 0.015625 based on rank 4 in query '1. "List of AI projects by Samarth"' Updating score for DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0.015873015873015872 to 0.032539682539682535 based on rank 0 in query '2. "Samarth AI projects portfolio"' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0.01639344262295082 to 0.03278688524590164 based on rank 1 in query '2. "Samarth AI projects portfolio"' Updating score for Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter.in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. It includes a custom-built hardware vending machine for immediate medicine dispensing Frequency Scaling + PWM Generator and 8 Bit UART Transmitter and Reciever iverilog implementation includes a frequency scaler with adjustable scaling factor and PWM generation based on a specified Duty Cycle. Additionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects Built a couple of Gen AI projects with a senior using preexisting diffusion models like StableDiffusionXL, InstantID, etc. for editing personalized video and cards. SmartGuardian www.gith ub.com/samarth777/smartguardian combines IoT with AI to create an autonomous car equipped with a virtual camera, allowing remote control and monitoring of surroundings with scene descriptions generated through AI integration MANET Research (ongoing) Researching Mobile Ad-hoc Networks under Dr. DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0.015625 to 0.031754032258064516 based on rank 2 in query '2. "Samarth AI projects portfolio"' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0.016666666666666666 to 0.032539682539682535 based on rank 3 in query '2. "Samarth AI projects portfolio"' Updating score for ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter. from 0.016129032258064516 to 0.031754032258064516 based on rank 4 in query '2. "Samarth AI projects portfolio"' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0.032539682539682535 to 0.0492063492063492 based on rank 0 in query '3. "Samarth artificial intelligence projects"' Updating score for DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0.032539682539682535 to 0.04893312516263336 based on rank 1 in query '3. "Samarth artificial intelligence projects"' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0.03278688524590164 to 0.04891591750396616 based on rank 2 in query '3. "Samarth artificial intelligence projects"' Updating score for ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter. from 0.031754032258064516 to 0.04762704813108039 based on rank 3 in query '3. "Samarth artificial intelligence projects"' Updating score for Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter.in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. It includes a custom-built hardware vending machine for immediate medicine dispensing Frequency Scaling + PWM Generator and 8 Bit UART Transmitter and Reciever iverilog implementation includes a frequency scaler with adjustable scaling factor and PWM generation based on a specified Duty Cycle. Additionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects Built a couple of Gen AI projects with a senior using preexisting diffusion models like StableDiffusionXL, InstantID, etc. for editing personalized video and cards. SmartGuardian www.gith ub.com/samarth777/smartguardian combines IoT with AI to create an autonomous car equipped with a virtual camera, allowing remote control and monitoring of surroundings with scene descriptions generated through AI integration MANET Research (ongoing) Researching Mobile Ad-hoc Networks under Dr. DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0.031754032258064516 to 0.047379032258064516 based on rank 4 in query '3. "Samarth artificial intelligence projects"' Updating score for Certifications/CoursesSA M A RTH P 3rd Year, B. Tech CSE at PES University, EC Campus Skills Proficient in Python, C, C++, JavaScript In depth knowledge of Machine learning Algorithms, GenAI, LLMs, RAG, Agentic AI Systems, Diffusion Models Full stack deveopment with Flutter, HTML, CSS, JavaScript, React Node.js, Next.js, MongoDB, Firebase, Flask, Django Experience in IoT and robotics, deep understanding of embedded systems notably Arduino Foundational understanding of Quantum Physics principles and practical experience using Qiskit for Quantum Computing Skilled in fine arts especially acrylic and watercolor paintings Education Background B Tech CSE, PES University EC Campus 2022 - Present | 8.56 CGPA GEAR Innovative International School Chinmaya Vidyalaya, Koramangala 2010 - 2020 | Grade X ICSE - 93%Profile samarthprakash8@gmail.com https://samarth.arthttps://github.com/samarth777 +91 7337610771 2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA 2nd Sem | 8.82 SGPA 3rd Sem | 8.63 SGPA Awarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at Arithemania’22 a 36-hr hackathon organised by Shunya Math Club + IEEE PESU Unimate'22 | Winner Team won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS PESU ECC Binary Battles | 3rd Place Won 3rd prize in this competitive coding event by Codechef PESU ECC Qiskit Quantum Challenge Spring 2023 Completed all labs in this IBM run event to test Qiskit knowledge Labyrinth Speed Coding Contest | 2nd Place Team won 2nd place in the speed coding challenge called Labyrinth organised by Codechef PESU ECC Codeventure | Winner Team won 1st place in the Codeventure Digital Quest organised by ACM-W PESUIBM Quantum Challenge 2024 Completed all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai CTF-Workshop and Hackathon, PESU C-ISFCR Certificate of Recognigition -HPE CodeWars 2022 Certificate of Recognigition -HPE CodeWars 2021 The Complete 2023 Web Development Bootcamp Workshop on “Various Aspects of Quantum Computing” -IIITB COMET Qiskit Global Summer School 2023 Dive into Deep Learning -d2l.ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. from 0.0492063492063492 to 0.06587301587301586 based on rank 0 in query '4. "AI initiatives by Samarth"' Updating score for com/in/samarth- p-3964721b3/Experience Summer Intern, IIIT Bangalore (June 2024 - Present) Working as a summer intern at the Web Science Lab (WSL), IIIT Bangalore under Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the project by implementing a pipeline to easily search information from a corpus in colloquial indic languages. Bosch University Connect Program (March 2024 - Present) Developing a Generative AI platform Bosch through the University Connect Program to help Automobile Technicians with Vehicular Troubleshoot Assistance Achievements Override'22 | Winner Team won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised by GDSC – PESU ECC Arithemania'22 | Winner RAS Track Team won 1st place at from 0.04891591750396616 to 0.06530936012691697 based on rank 1 in query '4. "AI initiatives by Samarth"' Updating score for DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0.04893312516263336 to 0.06506215742069787 based on rank 2 in query '4. "AI initiatives by Samarth"' Updating score for ai (currently doing) AMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category Idea awarded the AMD Kria KR260 FPGA for the development of an AI vision guided autonomous navigaing robot Clubs Quantumania PESU ECC -Club Secretary Built website for QuaNaD Lab, Conducted a workshop “Beyond Bits” explaining the fundamentals of Quantum Computing, Quantum research with Prof. Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter. from 0.04762704813108039 to 0.06350006400409626 based on rank 3 in query '4. "AI initiatives by Samarth"' Updating score for Gajanan Honnavar. Gave a lab tour and lecture for the QuaNaD Lab on the occasion of world Quantum Day Codechef PESU ECC -CP Mentor Speaker at a workshop that introduced 1st and 2nd year students to the C++ STL library Equinox PESU ECC -Technical Team IEEE RAS PESU ECC -Technical TeamProjects Handwriter https://handwriter.in Built a website that converts any typed piece of text into your own handwriting easily Gita Daily https://gitadaily.in Built a WhatsApp bot that sends subscribed users a verse from the Bhagavad Gita everyday (1000+ users) Websited for PESU Research Centres: QuaNaD, CONECT https://quanad.pes.edu Built a website for the Quantum and Nano Devices Laboratory of PESU ECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently building a website for Center for Networking and Evolving Communication Technologies CONECT) and a website for PESU to use for assignment submissions of various lab courses MedMaster www.github.com/samarth777/MedMaster An automated micro-pharmacy facilitates quick medicine purchases through QR scanning on our website. It includes a custom-built hardware vending machine for immediate medicine dispensing Frequency Scaling + PWM Generator and 8 Bit UART Transmitter and Reciever iverilog implementation includes a frequency scaler with adjustable scaling factor and PWM generation based on a specified Duty Cycle. Additionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects Built a couple of Gen AI projects with a senior using preexisting diffusion models like StableDiffusionXL, InstantID, etc. for editing personalized video and cards. SmartGuardian www.gith ub.com/samarth777/smartguardian combines IoT with AI to create an autonomous car equipped with a virtual camera, allowing remote control and monitoring of surroundings with scene descriptions generated through AI integration MANET Research (ongoing) Researching Mobile Ad-hoc Networks under Dr. DP Chavan using tools like NS3Bosch University Connect Program -VTAGen AI Project (ongoing) Building a GenAI project for Bosch to help technicians with vehicular troublehoot assistance by creating a platform powered by RAG and finetuned LLM’sAlienWear https://alienwear.vercel.app An AI-powered fashion e-commerce platform that revolutionizes clothing discovery and purchasing. It uses RAG and vector search for semantic queries, enabling users to find products using natural languageKissanDial https://github.com/shadow-penguins/-KissanDial A voice call-based AI agent assistant designed to empower farmers by providing them with vital information on agricultural subsidies, weather updates, and more. By addressing key challenges faced by farmers KissanDial aims to enhance their access to essential resources. from 0.047379032258064516 to 0.06300403225806452 based on rank 4 in query '4. "AI initiatives by Samarth"' Final reranked results: {"Certifications/CoursesSA M A RTH P\n3rd Year, B. Tech CSE at PES University, EC Campus\nSkills\nProficient in Python, C, C++, JavaScript\nIn depth knowledge of Machine\nlearning Algorithms, GenAI, LLMs,\nRAG, Agentic AI Systems, Diffusion\nModels\nFull stack deveopment with Flutter,\nHTML, CSS, JavaScript, React Node.js,\nNext.js, MongoDB, Firebase, Flask,\nDjango\nExperience in IoT and robotics, deep\nunderstanding of embedded systems\nnotably Arduino\nFoundational understanding of\nQuantum Physics principles and\npractical experience using Qiskit for\nQuantum Computing \nSkilled in fine arts especially acrylic\nand watercolor paintings\nEducation Background\nB Tech CSE, PES University EC Campus\n2022 - Present | 8.56 CGPA\nGEAR Innovative International School\nChinmaya Vidyalaya, Koramangala\n2010 - 2020 | Grade X ICSE - 93%Profile\nsamarthprakash8@gmail.com\nhttps://samarth.arthttps://github.com/samarth777\n+91 7337610771\n2020 - 2022 | Grade XII CBSE - 87%1st Sem | 8.23 SGPA\n2nd Sem | 8.82 SGPA\n3rd Sem | 8.63 SGPA\nAwarded CNR Scholarship in Semester 3https://www.linkedin.com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at Arithemania’22 a 36-hr hackathon organised by\nShunya Math Club + IEEE PESU \nUnimate'22 | Winner \nTeam won 1st place at Unimate’22 a 24-hr hackathon organised by IEEE RAS\nPESU ECC \nBinary Battles | 3rd Place\nWon 3rd prize in this competitive coding event by Codechef PESU ECC\nQiskit Quantum Challenge Spring 2023\nCompleted all labs in this IBM run event to test Qiskit knowledge\nLabyrinth Speed Coding Contest | 2nd Place\nTeam won 2nd place in the speed coding challenge called Labyrinth\norganised by Codechef PESU ECC\nCodeventure | Winner\nTeam won 1st place in the Codeventure Digital Quest organised by ACM-W\nPESUIBM Quantum Challenge 2024\nCompleted all labs in this IBM event working with the new Qiskit 1.0 SDKMachine Learning Specialization - deeplearning.ai\nCTF-Workshop and Hackathon, PESU C-ISFCR\nCertificate of Recognigition -HPE CodeWars 2022\nCertificate of Recognigition -HPE CodeWars 2021\nThe Complete 2023 Web Development Bootcamp\nWorkshop on “Various Aspects of Quantum Computing” -IIITB COMET\nQiskit Global Summer School 2023\nDive into Deep Learning -d2l.ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.": 0.06587301587301586, "com/in/samarth-\np-3964721b3/Experience\nSummer Intern, IIIT Bangalore (June 2024 - Present)\nWorking as a summer intern at the Web Science Lab (WSL), IIIT Bangalore\nunder Prof. Srinath Srinivasa on the IndicNLP project. Contributing to the\nproject by implementing a pipeline to easily search information from a\ncorpus in colloquial indic languages.\nBosch University Connect Program (March 2024 - Present)\nDeveloping a Generative AI platform Bosch through the University\nConnect Program to help Automobile Technicians with Vehicular\nTroubleshoot Assistance\nAchievements\nOverride'22 | Winner\nTeam won ‘Best Overall App’ at Override ’22 a 48-hr hackathon organised\nby GDSC – PESU ECC\nArithemania'22 | Winner RAS Track\nTeam won 1st place at": 0.06530936012691697, 'DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.06506215742069787, 'ai (currently doing)\nAMD Pervasive AI Hackathon | Hardware Winner Robotics AI Category\nIdea awarded the AMD Kria KR260 FPGA for the development of an AI vision\nguided autonomous navigaing robot\n\nClubs\nQuantumania PESU ECC -Club\nSecretary Built website for QuaNaD Lab,\nConducted a workshop “Beyond Bits”\nexplaining the fundamentals of\nQuantum Computing, Quantum\nresearch with Prof. Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.': 0.06350006400409626, 'Gajanan Honnavar.\nGave a lab tour and lecture for the\nQuaNaD Lab on the occasion of world\nQuantum Day\nCodechef PESU ECC -CP Mentor \nSpeaker at a workshop that introduced\n1st and 2nd year students to the C++ STL\nlibrary\nEquinox PESU ECC -Technical Team\nIEEE RAS PESU ECC -Technical TeamProjects\nHandwriter https://handwriter.in\nBuilt a website that converts any typed piece of text into your own\nhandwriting easily\nGita Daily https://gitadaily.in\nBuilt a WhatsApp bot that sends subscribed users a verse from the\nBhagavad Gita everyday (1000+ users)\nWebsited for PESU Research Centres: QuaNaD, CONECT \nhttps://quanad.pes.edu\nBuilt a website for the Quantum and Nano Devices Laboratory of PESU\nECC using Next.js 13 and Tailwind CSS hosted on Vercel. Currently\nbuilding a website for Center for Networking and Evolving\nCommunication Technologies CONECT) and a website for PESU to use for\nassignment submissions of various lab courses\nMedMaster www.github.com/samarth777/MedMaster\nAn automated micro-pharmacy facilitates quick medicine purchases\nthrough QR scanning on our website. It includes a custom-built\nhardware vending machine for immediate medicine dispensing\nFrequency Scaling + PWM Generator and 8 Bit UART Transmitter and\nReciever\niverilog implementation includes a frequency scaler with adjustable\nscaling factor and PWM generation based on a specified Duty Cycle.\nAdditionally, an 8-bit UART transmitter and receiver are integrated.Generative AI Projects\nBuilt a couple of Gen AI projects with a senior using preexisting diffusion\nmodels like StableDiffusionXL, InstantID, etc. for editing personalized\nvideo and cards.\nSmartGuardian www.gith ub.com/samarth777/smartguardian\ncombines IoT with AI to create an autonomous car equipped with a\nvirtual camera, allowing remote control and monitoring of surroundings\nwith scene descriptions generated through AI integration\nMANET Research (ongoing)\nResearching Mobile Ad-hoc Networks under Dr. DP Chavan using tools\nlike NS3Bosch University Connect Program -VTAGen AI Project (ongoing)\nBuilding a GenAI project for Bosch to help technicians with vehicular\ntroublehoot assistance by creating a platform powered by RAG and\nfinetuned LLM’sAlienWear https://alienwear.vercel.app\nAn AI-powered fashion e-commerce platform that revolutionizes\nclothing discovery and purchasing. It uses RAG and vector search for\nsemantic queries, enabling users to find products using natural\nlanguageKissanDial https://github.com/shadow-penguins/-KissanDial\nA voice call-based AI agent assistant designed to empower farmers by\nproviding them with vital information on agricultural subsidies, weather\nupdates, and more. By addressing key challenges faced by farmers\nKissanDial aims to enhance their access to essential resources.': 0.06300403225806452}
Human: What are all the AI projects done by samarth?
AI: The AI projects done by Samarth are:
- Gen AI Project for Bosch under the University Connect Program
- AlienWear - AI-powered fashion e-commerce platform
- KissanDial - Voice call-based AI agent assistant
--------------------------------------------------
'The AI projects done by Samarth are:\n\n1. Gen AI Project for Bosch under the University Connect Program\n2. AlienWear - AI-powered fashion e-commerce platform\n3. KissanDial - Voice call-based AI agent assistant'
# To view chat history:
history = chat_interface.get_chat_history()
for message in history:
print(f"{message.role}: {message.content}")
MessageRole.USER: What is Samarth's CGPA? MessageRole.ASSISTANT: Samarth's CGPA is 8.56 at PES University EC Campus where he is pursuing B. Tech CSE from 2022 to present. MessageRole.USER: What are all the AI projects done by samarth? MessageRole.ASSISTANT: The AI projects done by Samarth are: 1. Gen AI Project for Bosch under the University Connect Program 2. AlienWear - AI-powered fashion e-commerce platform 3. KissanDial - Voice call-based AI agent assistant